Send and Track Events
This document will cover the method to send event to Suprsend platform
Pre-requisites
- Integrate Go SDK
- Create User Profile
- Create Template on SuprSend platform - if you want to trigger workflow by passing the event
- Create Workflow on SuprSend Platform - if you want to trigger workflow by passing the event
Create Workflow on SuprSend Platform
For Event based workflow trigger, you'll have to create the workflow on SuprSend Platform. Once the workflow is created, you can pass the EventName
( GROCERY_PURCHASED
in below example) defined in workflow configuration with the help of suprClient.TrackEvent
method. Variables added in the template should be passed as event Properties
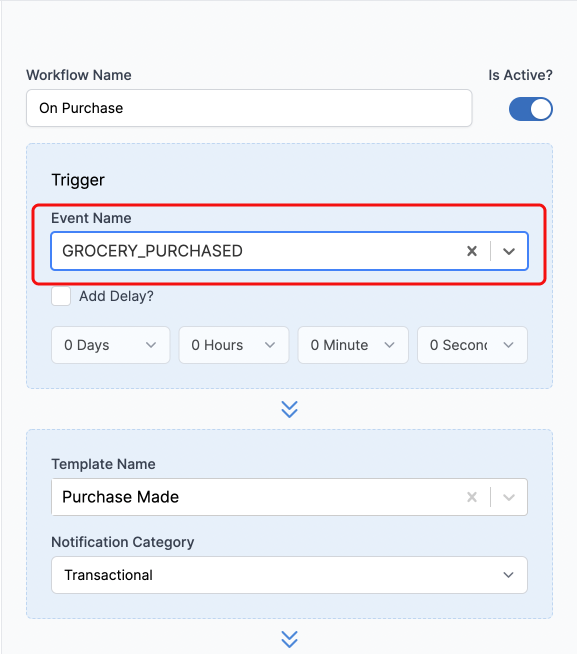
Send Event
You can send event to Suprsend platform by using the suprClient.TrackEvent
method.
ev := &suprsend.Event{
EventName: "__event_name__",
DistinctId: "__distinct_id__",
Properties: map[string]interface{}{
"k1": "v1",
},
IdempotencyKey: "123456"
}
// Add attachment (If needed) by calling .AddAttachment
err = ev.AddAttachment("~/Downloads/attachment.pdf", &suprsend.AttachmentOption{FileName: "My Attachment.pdf"})
if err != nil {
log.Println(err)
}
// Send event to Suprsend by calling .TrackEvent
_, err = suprClient.TrackEvent(ev)
if err != nil {
log.Fatalln(err)
}
Parameter | Description | Type | Obligation |
---|---|---|---|
DistinctId | distinct_id of user performing the event | string | mandatory |
EventName | string identifier for the event like product_purchased | string | mandatory |
Properties | a dictionary representing event attributes like first_name Event properties can be used to pass template variables in case of event based trigger | Dictionary | optional |
IdempotencyKey | idempotency_key is the unique identifier for an event, and expires in 24 hours. | string | optional |
Event naming guidelines
When you create an Event or a property, please ensure that the Event Name or Property Name does not start with
$
orss_
, as we have reserved these symbols for our internal events and property names.
Sample Code
ev := &suprsend.Event{
EventName: "product_purchased", // Mandatory, name of the event you're tracking
DistinctId: "0fxxx8f74-xxxx-41c5-8752-xxxcb6911fb08", // Mandatory, Unique id of user in your application
Properties: map[string]interface{}{
"first_name": "User",
"spend_amount": "$10",
"nested_key_example": map[string]interface{}{
"nested_key1": "some_value_1",
"nested_key2": map[string]interface{}{
"nested_key3": "some_value_3",
},
},
IdempotencyKey: "abcd-1234-abc6"
}
// Add attachment (If needed) by calling .AddAttachment
err = ev.AddAttachment("~/Downloads/attachment.pdf", &suprsend.AttachmentOption{FileName: "My Attachment.pdf"})
if err != nil {
log.Println(err)
}
// Send event to Suprsend by calling .TrackEvent
_, err = suprClient.TrackEvent(ev)
if err != nil {
log.Fatalln(err)
}
Note
EventName in Track event method should exactly match the Event name added in Workflow configurations
Response
When you call suprClient.TrackEvent
, the SDK internally makes an HTTP
call to SuprSend Platform to register this request, and you'll receive an error message if something fails
Bulk API for multiple event requests
You can use Bulk API to send multiple events.
Use .Append()
on bulk_events instance to add however-many-records to call in bulk.
ev1 := &suprsend.Event{
EventName: "__event_name__",
DistinctId: "__distinct_id__",
Properties: map[string]interface{}{
"k1": "v1",
},
IdempotencyKey: ""
}
ev2 := &suprsend.Event{
EventName: "__event_name__",
DistinctId: "__distinct_id__",
IdempotencyKey: ""
}
// Create bulkEvents instance
bulkIns := suprClient.BulkEvents.NewInstance()
// Add all events to bulk Instance
bulkIns.Append(ev1, ev2)
// call trigger to send all these events to SuprSend
bulkResponse, err := bulkIns.Trigger()
if err != nil {
log.Fatalln(err)
}
log.Println(bulkResponse)
How SuprSend Processes the bulk API request
- On calling
bulkIns.Trigger()
, the SDK internally makes one-or-more Callable-chunks. - Each callable-chunk contains a subset of records, the subset calculation is based on each record's bytes-size and max allowed chunk-size, chunk-length etc.
- For each callable-chunk SDK makes an HTTP call to SuprSend to register the request.
Response
Response is an instance of suprsend.BulkResponse
type
// Response structure
suprsend.BulkResponse{
Status : "success",
Total : 10, // number of records sent in bulk
Success : 10, // number of records succeeded
Failure : 0, // number of records failed
FailedRecords : [],
}
suprsend.BulkResponse{
Status : "fail",
Total : 10, // number of records sent in bulk
Success : 0, // number of records succeeded
Failure : 10, // number of records failed
FailedRecords : [{"record": {...}, "error": "error_str", "code": 500}]
}
suprsend.BulkResponse{
Status : "partial",
Total : 10, // number of records sent in bulk
Success : 6, // number of records succeeded
Failure : 4, // number of records failed
FailedRecords : [{"record": {...}, "error": "error_str", "code": 500}]
}
Updated about 1 year ago